● 좋아요 및 댓글작성, 댓글창
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
</head>
<body>
<button id='like'>좋아요</button>
<span>0</span>
<br>
<input type="text">
<button class='writeCom'>댓글작성</button>
<br>
<div id="com">
<p>첫댓글<button class='removeCom'>삭제</button></p>
</div>
<script>
// 1,2 => on('click')사용
// 1. '좋아요' 버튼을 눌렀을 때, 0->1 / 좋아요 -> 좋아요 취소
// => id를 dislike로 변경
// 2. '좋아요 취소' 버튼을 눌렀을 때, 1-> 0 / 좋아요 취소 -> 좋아요
// => id를 like로 변경
// 3. 댓글을 작성
// 내가 적은 댓글 + 삭제버튼
// 댓글을 작성한 후 => input창은 비워줄것!
// 삭제 버튼 => 내가 삭제한 '그 댓글'만 지워줄 것!
$(document).on('click','#like',function(){
//좋아요 0->1
$('span').text('1')
//좋아요 -> 좋아요 취소 (ex03->3번)
$(this).text('좋아요 취소')
//id라는 속성삭제
$(this).removeAttr('id')
//버튼에 dislike라는 id부여
$(this).attr('id','dislike')
})
$(document).on('click','#dislike',function(){
//좋아요 1->0
$('span').text('0')
//좋아요 -> 좋아요 취소 (ex03->3번)
$(this).text('좋아요')
// id라는 속성삭제
$(this).removeAttr('id')
//버튼에 dislike라는 id부여
$(this).attr('id','like')
})
$('.writeCom').click(()=>{
let com = $('input[type=text]').val()
$('#com').prepend("<p>"+com+"<button class='removeCom'>삭제</button></p>")
$('input[type=text]').val("")
})
$(document).on('click','.removeCom',function(){
$(this).parent().remove()
})
</script>
</body>
</html>
<head> 부분
<script src="https://code.jquery.com/jquery-3.6.0.min.js"> # jQuery를 사용하겠다고 선언
<body> 부분
<button id='like'>좋아요</button>
<span>0</span>
<br>
<input type="text">
<button class='writeCom'>댓글작성</button>
<br>
<div id="com">
<p>첫댓글<button class='removeCom'>삭제</button></p>
</div>
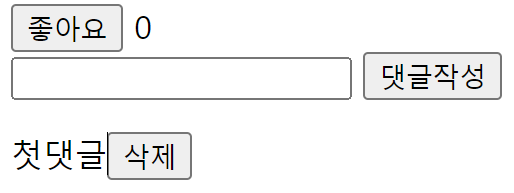
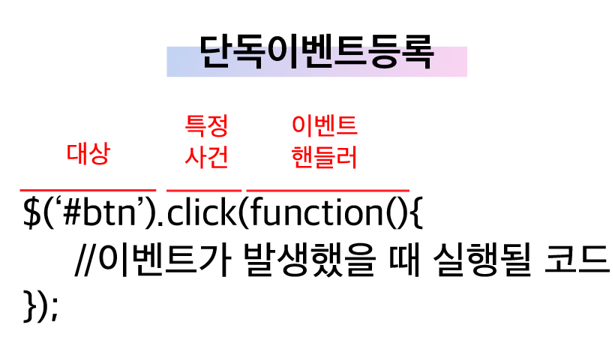
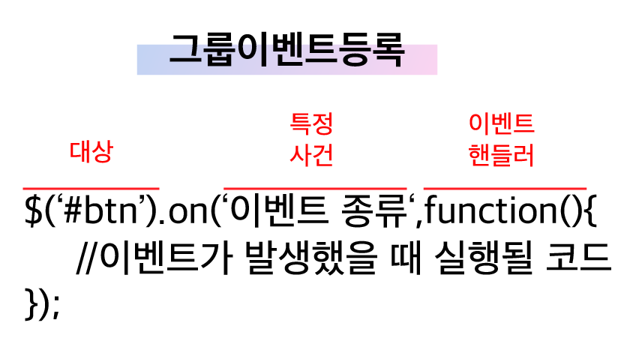

.click vs .on('click')
동적 이벤트 바인딩 차이
.click : 최초의 페이지를 로딩할 때 선언되어있던
요소들에 이벤트를 바인딩 그 이후 더이상 바인딩하지 않음
on('click'): 동적으로 추가된 태그 이벤트 바인딩
1. '좋아요' 버튼을 눌렀을 때, 0->1 / 좋아요 -> 좋아요 취소
=> id를 dislike로 변경
$(document).on('click','#like',function(){ # document에서 #like라는 id의 button를 클릭했을 때
//좋아요 0->1
$('span').text('1') # span태그를 1로 변경
//좋아요 -> 좋아요 취소 (ex03->3번)
$(this).text('좋아요 취소') # 위에서 실행한 #like라는 id의 좋아요를 좋아요 취소로 변경
//id라는 속성삭제
$().removeAttr() # id의 속성을 제거한다.
//버튼에 dislike라는 id부여
$(this).attr('id','dislike') # 다시 이 버튼에 id에 dislike라는 속성을 준다.
})
결과)

2. '좋아요 취소' 버튼을 눌렀을 때, 1-> 0 / 좋아요 취소 -> 좋아요
=> id를 like로 변경
$(document).on('click','#dislike',function(){ # document에서 #disike라는 id의 button를 클릭했을 때
//좋아요 1->0
$('span').text('0') # span태그를 0으로 변경
//좋아요 -> 좋아요 취소 (ex03->3번)
$(this).text('좋아요') # 위에서 실행한 #dislike라는 id의 좋아요 취소를 좋아요로 변경
// id라는 속성삭제
$(this).removeAttr('id') # id의 속성을 제거한다.
//버튼에 dislike라는 id부여
$(this).attr('id','like') # 다시 이 버튼에 id에 like라는 속성을 준다.
})
결과)

▼
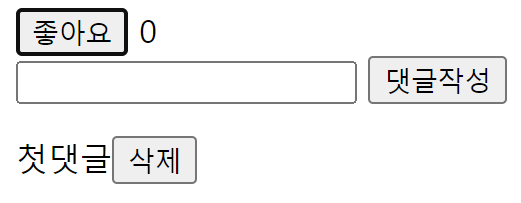
3. 댓글을 작성
내가 적은 댓글 + 삭제버튼
댓글을 작성한 후 => input창은 비워줄것!
삭제 버튼 => 내가 삭제한 '그 댓글'만 지워줄 것!
$('.writeCom').click(()=>{ # writeCom class의 버튼을 눌렀을때
let com = $('input[type=text]').val() # [type=text]인 input 요소의 값을 변수 com에 저장
$('#com').prepend("<p>"+com+"<button class='removeCom'>삭제</button></p>")
# com class의 앞에 변수 com에 저장된 input 값뒤에 class가 removeCom인 class "삭제"버튼을 추가
$('input[type=text]').val("") # 댓글을 작성한 후 input창은 비워줌 -> ("")!
})
$(document).on('click','.removeCom',function(){ # removeCom이라는 class를 눌렀을때
$(this).parent().remove() # removeCom class의 버튼의 부모를 삭제함
})
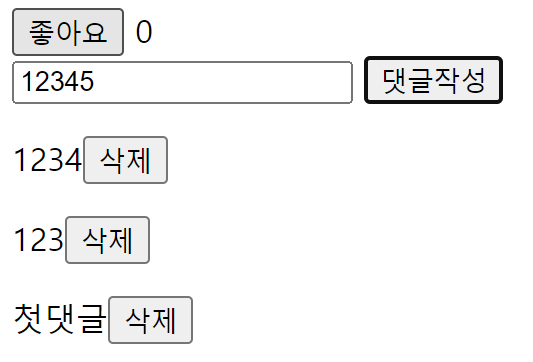
▼
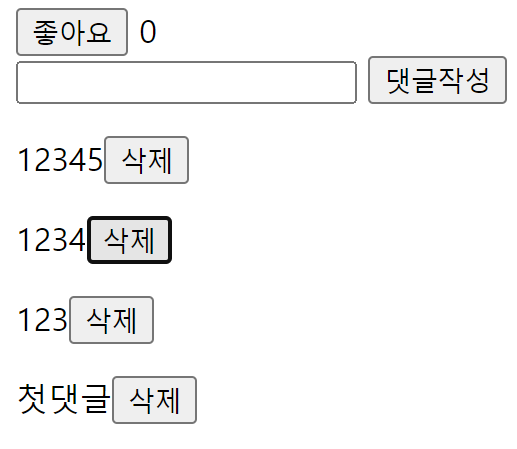
▼

'개발 공부 > JS, JQuery' 카테고리의 다른 글
Jquery(제이쿼리) 차트만들기, Ajax를 이용해 날씨차트만들기 (1) | 2023.10.24 |
---|---|
Jquery(제이쿼리) 오픈API, Ajax방식으로 데이터를 요청해 json데이터 추출(영화, 지도) (1) | 2023.10.24 |
자바스크립트 this 4가지 사용법 (0) | 2023.10.19 |
자바스크립트 로그인 폼 실습, 요소 추가 삭제 함수 (0) | 2023.10.19 |
자바스크립트 마우스 이벤트 (0) | 2023.10.19 |